In this post, we will see how to deploy a Spring Boot application on an external tomcat server. We are going to use Apache Tomcat 8 (i.e apache-tomcat-8.5.75) for this tutorial. If you are a beginner, it is highly recommended to follow the same step. We are going to use the spring boot 2.3.1 release. First, we will create war using maven then we will deploy it on an external tomcat. We are going to follow the below steps to deploy the Spring Boot application on an external tomcat.
Note – Source code download link has been given at the end of the tutorial.
- Add dependency in pom.xml to exclude embedded tomcat so that we can further deploy our war file on external tomcat.
- Define SpringMain class by extending SpringBootServletInitializer class and override configure() method.
- Create Student.java and StudentController class.
- Build the application using maven build command.
- Download the tomcat and extract it.
- Keep the war file in webapps folder.
- Run the startup.bat file.
- Test the application.
Note – Since we are going to deploy our spring boot application on external tomcat application.properties file is not mandatory.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
2. Define SpringMain class by extending SpringBootServletInitializer class and override configure() method. In order to use SpringBootServletInitializer we need to add javax.servlet dependency explicitly.
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<scope>provided</scope>
</dependency>
SpringMain.java
@SpringBootApplication
public class SpringMain extends SpringBootServletInitializer {
public static void main(String[] args) {
SpringApplication.run(SpringMain.class, args);
}
@Override
public SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(SpringMain.class);
}
}
3. Create Student.java and StudentController class. This class contains the rest endpoint(i.e http://localhost:8283/deployusingexternaltomcat/simpleuri ).
Note – In pom.xml we need to provide a war name. In this example, we will give the war name deployusingexternaltomcat in pom.xml.
public class Student {
private Long id;
private String name;
private String rollNumber;
//getter & setter
}
StudentController.java
@RestController
class BookController {
@RequestMapping(value = "/simpleuri", method = RequestMethod.GET)
@ResponseBody
public Student getStudent() {
Student student = new Student();
student.setId(1l);
student.setRollNumber("0126CS071");
student.setName("rakesh");
return student;
}
}
Note – See more details about @RestController annotation.
4. Build the application using the maven build command(i.e mvn clean install). In the target folder, the war file should get created.
We can create a war file using a different way. Sometimes people use eclipse itself to build the application, also we can use the command prompt. Here we will use git bash for the build.
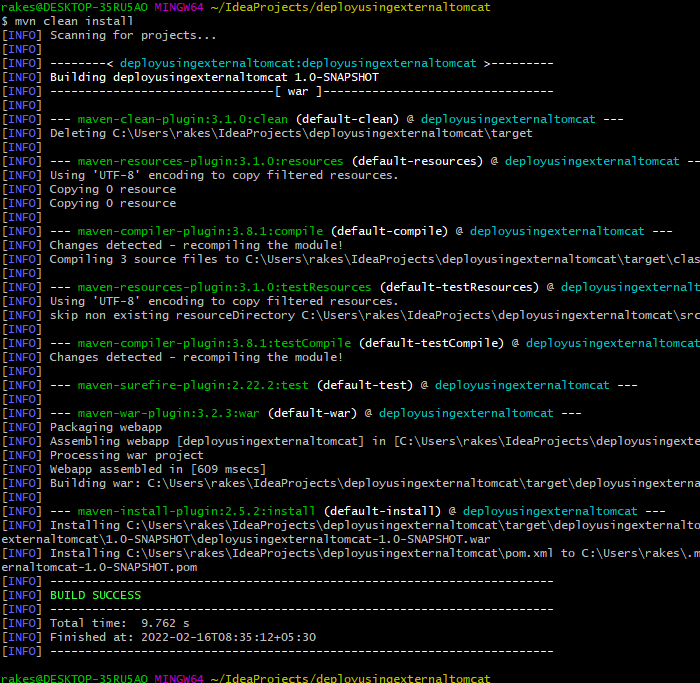
We should be able to war file in the target folder.

5. Download the tomcat and extract it. Change the deployment port in server.xml if needed.

Note – If you see Caused by: java.net.BindException: Address already in use: bind exception, change the port to 8283 or something else than 8080.

6. Copy the war file from the target folder and keep it into apache-tomcat-8.5.75\webapps directory and rename the war file as below.
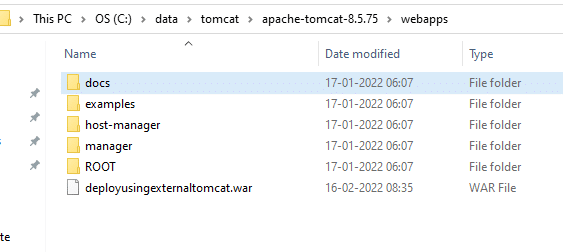
7. Go to Tomcat’s bin folder and Run the startup.bat file. Our tomcat should be up and running.

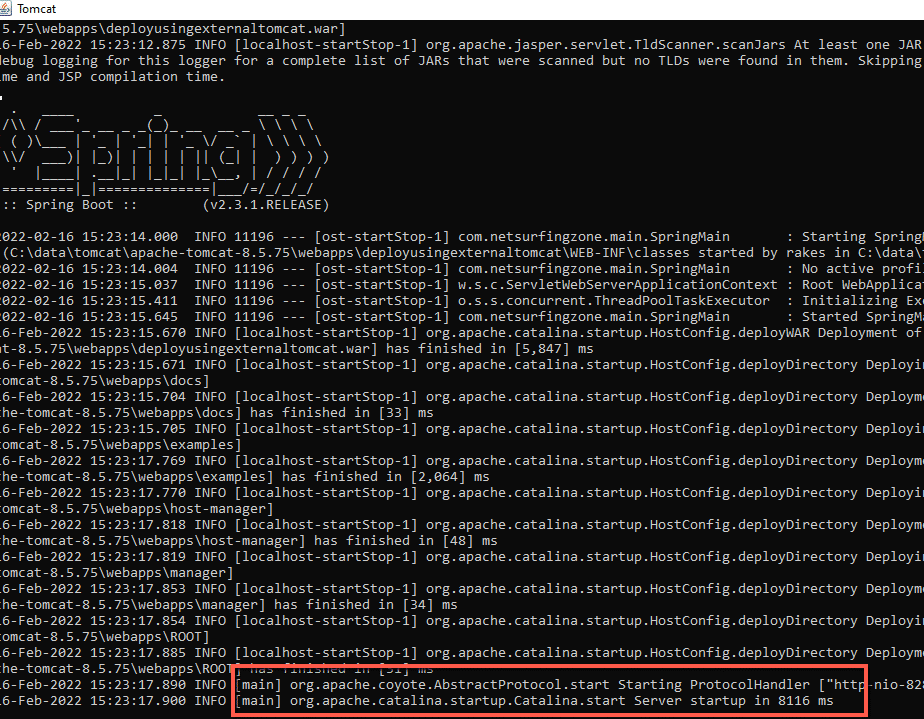
8. Test the application using http://localhost:8283/deployusingexternaltomcat/simpleuri
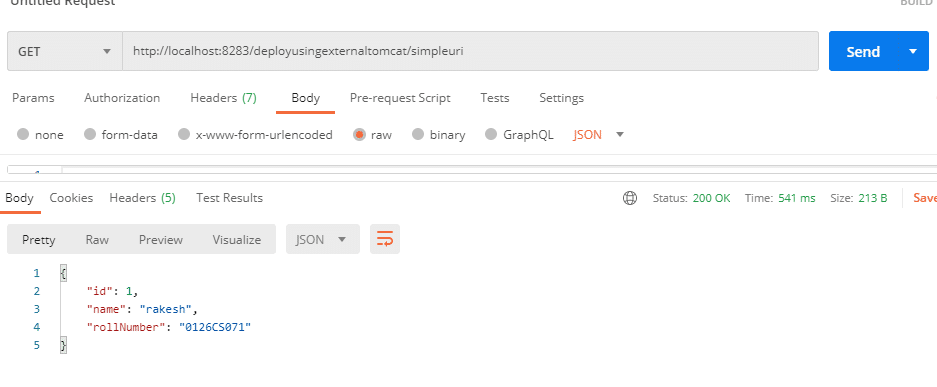
Let’s see the complete example for the Deploy Spring Boot application on external Tomcat.
Create maven project
Directory structure

Define pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>deployusingexternaltomcat</groupId>
<artifactId>deployusingexternaltomcat</artifactId>
<version>1.0-SNAPSHOT</version>
<name>deployusingexternaltomcat</name>
<packaging>war</packaging>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.1.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
</project>
Define model class Student.java
package com.javatute.entity;
public class Student {
private Long id;
private String name;
private String rollNumber;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getRollNumber() {
return rollNumber;
}
public void setRollNumber(String rollNumber) {
this.rollNumber = rollNumber;
}
}
Define controller class
package com.javatute;
import com.javatute.entity.Student;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
class BookController {
@RequestMapping(value = "/simpleuri", method = RequestMethod.GET)
@ResponseBody
public Student getBook() {
Student student = new Student();
student.setId(1l);
student.setRollNumber("0126CS071");
student.setName("rakesh");
return student;
}
}
Define SpringMain.java class
package com.javatute.main;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
import org.springframework.context.annotation.ComponentScan;
@ComponentScan(basePackages = "com.javatute")
@SpringBootApplication
public class SpringMain extends SpringBootServletInitializer {
public static void main(String[] args) {
SpringApplication.run(SpringMain.class, args);
}
@Override
public SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(SpringMain.class);
}
}
That’s all about how to Deploy Spring Boot application on external Tomcat. If you face any issues please leave a comment.
Download source code from Github.
Apache Tomcat docs.
Spring boot Datasource configuration using tomcat
Deploy Spring boot war in JBoss EAP server.
Jboss 7 EPA Datasource configuration using oracle and spring boot.
Deploy multiple war files in JBoss to Different port.