In this tutorial, we will see JsonItemReader Spring Batch Example. We will see how to read JSON file in the spring batch using JsonItemReader class and store it in the MySql database. The JsonItemReader class uses JsonObjectReader.
Note – The JsonItemReader implementation is not thread safe. It supports the below format.
[ { JSON object }, { JSON object } ]
Consider we have the following JSON file in the resource directory.
[{
"id": 1,
"name": "John",
"rollNumber": "0126CS090"
}, {
"id": 2,
"name": "Arya",
"rollNumber": "0126CS090"
}, {
"id": 3,
"name": "Will",
"rollNumber": "0126CS090"
}, {
"id": 4,
"name": "Smith",
"rollNumber": "0126CS090"
}, {
"id": 5,
"name": "Rock",
"rollNumber": "0126CS090"
}]
We will read these JSON records using JsonItemReader and store them in the MySql database.

Configuring JsonItemReader.
public JsonItemReader<Student> jsonItemReader() {
return new JsonItemReaderBuilder<Student>()
.jsonObjectReader(new JacksonJsonObjectReader<>(Student.class))
.resource(new ClassPathResource("data.json"))
.name("studentJsonItemReader")
.build();
}
Let’s see Spring Batch JsonItemReader Example from scratch.
Maven dependency
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.javatute.com</groupId>
<artifactId>springbatchjsonitemreader</artifactId>
<version>1.0-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.1.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.batch</groupId>
<artifactId>spring-batch-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-batch</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
</dependencies>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
</project>
Directory structure

Define Student.java
package com.springbatchexample.entity;
public class Student {
private Long id;
private String name;
private String rollNumber;
public Student() {
}
public Student(Long id, String name, String rollNumber) {
this.id = id;
this.name = name;
this.rollNumber = rollNumber;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getRollNumber() {
return rollNumber;
}
public void setRollNumber(String rollNumber) {
this.rollNumber = rollNumber;
}
}
Define StudentItemProcessor
import com.springbatchexample.entity.Student;
import org.springframework.batch.item.ItemProcessor;
public class StudentItemProcessor implements ItemProcessor<Student, Student> {
@Override
public Student process(Student student) throws Exception {
return student;
}
}
The StudentItemProcessor can be used to perform any transformations or validation that we need on an item(i.e student) before Spring Batch sends it to the ItemWriter. we are not performing transformations or validation for item.
Define SpringBatchConfig file
package com.springbatchexample.config;
import com.springbatchexample.entity.Student;
import org.springframework.batch.core.Job;
import org.springframework.batch.core.Step;
import org.springframework.batch.core.configuration.annotation.EnableBatchProcessing;
import org.springframework.batch.core.configuration.annotation.JobBuilderFactory;
import org.springframework.batch.core.configuration.annotation.StepBuilderFactory;
import org.springframework.batch.core.job.builder.FlowJobBuilder;
import org.springframework.batch.core.job.builder.JobBuilder;
import org.springframework.batch.core.launch.support.RunIdIncrementer;
import org.springframework.batch.core.step.builder.SimpleStepBuilder;
import org.springframework.batch.core.step.builder.StepBuilder;
import org.springframework.batch.item.database.BeanPropertyItemSqlParameterSourceProvider;
import org.springframework.batch.item.database.JdbcBatchItemWriter;
import org.springframework.batch.item.file.FlatFileItemReader;
import org.springframework.batch.item.file.LineMapper;
import org.springframework.batch.item.file.mapping.BeanWrapperFieldSetMapper;
import org.springframework.batch.item.file.mapping.DefaultLineMapper;
import org.springframework.batch.item.file.transform.DelimitedLineTokenizer;
import org.springframework.batch.item.json.JacksonJsonObjectReader;
import org.springframework.batch.item.json.JsonItemReader;
import org.springframework.batch.item.json.builder.JsonItemReaderBuilder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.io.ClassPathResource;
import javax.sql.DataSource;
@EnableBatchProcessing
@Configuration
public class SpringBatchConfig {
@Autowired
private DataSource dataSource;
@Autowired
private JobBuilderFactory jobBuilderFactory;
@Autowired
private StepBuilderFactory stepBuilderFactory;
public JsonItemReader<Student> jsonItemReader() {
return new JsonItemReaderBuilder<Student>()
.jsonObjectReader(new JacksonJsonObjectReader<>(Student.class))
.resource(new ClassPathResource("data.json"))
.name("studentJsonItemReader")
.build();
}
@Bean
public StudentItemProcessor processor() {
return new StudentItemProcessor();
}
@Bean
public JdbcBatchItemWriter<Student> writer() {
JdbcBatchItemWriter<Student> writer = new JdbcBatchItemWriter<>();
writer.setItemSqlParameterSourceProvider(new BeanPropertyItemSqlParameterSourceProvider<>());
writer.setSql("insert into student(id,roll_number,name) values (:id,:rollNumber,:name)");
writer.setDataSource(dataSource);
return writer;
}
@Bean
public Job writeStudentDataIntoSqlDb() {
JobBuilder jobBuilder = jobBuilderFactory.get("STUDENT_JOB");
jobBuilder.incrementer(new RunIdIncrementer());
FlowJobBuilder flowJobBuilder = jobBuilder.flow(getFirstStep()).end();
Job job = flowJobBuilder.build();
return job;
}
@Bean
public Step getFirstStep() {
StepBuilder stepBuilder = stepBuilderFactory.get("getFirstStep");
SimpleStepBuilder<Student, Student> simpleStepBuilder = stepBuilder.chunk(1);
return simpleStepBuilder.reader(jsonItemReader()).processor(processor()).writer(writer()).build();
}
}
application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/springbootcrudexample
spring.datasource.username=root
spring.datasource.password=root
spring.jpa.hibernate.ddl-auto=create
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.format_sql=true
server.port = 9091
spring.batch.initialize-schema=always
Define SpringMain class
package com.springbatchexample.main;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.ComponentScan;
@SpringBootApplication
@ComponentScan(basePackages = "com.springbatchexample.*")
public class SpringMain {
public static void main(String[] args) {
SpringApplication.run(SpringMain.class, args);
}
}
Once we run the application we should be able to see data in the database.
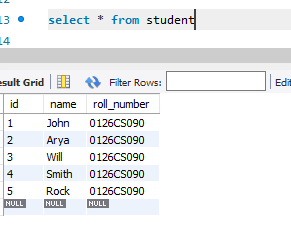
That’s all about JsonItemReader Spring Batch Example.
see docs for JsonItemReader.
Download source code from GitHub.
Spring Batch tutorials.
- Spring Batch FlatFileItemReader Example
- StoredProcedureItemReader example
- JdbcCursorItemReader Spring Batch Example
- StaxEventItemReader Example
Spring Data JPA examples
- Spring Data JPA greater than Example
- Spring Data JPA less than Example
- Spring Data JPA IsNull Example Using Spring Boot
- Spring Data findById() Vs getOne()
- Spring Data JPA CrudRepository findById()
- Spring Data JPA JpaRepository getOne()
- Spring Data CrudRepository saveAll() and findAll().
- Spring Data CrudRepository existsById()
- Spring Data JPA delete() vs deleteInBatch()
- Spring Data JPA deleteAll() Vs deleteAllInBatch()
- Spring Data JPA JpaRepository deleteAllInBatch()
- Spring Data JPA deleteInBatch() Example
- Spring Data JPA JpaRepository saveAndFlush() Example
- Spring Data JPA CrudRepository count() Example
- Spring Data JPA CrudRepository delete() and deleteAll()
- Spring Data JPA CrudRepository deleteById() Example
- CrudRepository findAllById() Example Using Spring Boot
- Spring Data CrudRepository save() Method.
- Sorting in Spring Data JPA using Spring Boot.
- Spring Data JPA example using spring boot.
- Spring Data JPA and its benefit.
Hibernate example using spring boot.
- @Version Annotation Example In Hibernate.
- Hibernate Validator Constraints Example Using Spring Boot.
- @Temporal Annotation Example In Hibernate/Jpa Using Spring Boot.
- Hibernate Table Per Concrete Class Spring Boot.
- Hibernate Table Per Subclass Inheritance Spring Boot.
- Hibernate Single Table Inheritance using Spring Boot.
- One To One Mapping Annotation Example in Hibernate/JPA using Spring Boot and Oracle.
- One To One Bidirectional Mapping Example In Hibernate/JPA Using Spring Boot and Oracle.
- One To Many Mapping Annotation Example In Hibernate/JPA Using Spring Boot And Oracle.
- Many To One Unidirectional Mapping In Hibernate/JPA Annotation Example Using Spring Boot and Oracle.
- One To Many Bidirectional Mapping In Hibernate/JPA Annotation Example Using Spring Boot and Oracle.
- Many To Many Mapping Annotation Example In Hibernate/JPA Using Spring Boot