In this post, we will see @PathVariable and @RequestParam annotations in Spring Boot. We will see how to use @RequestParam and @PathVariable to create Rest APIs. How both differ from each other. Consider we have a use case where we want to retrieve the book for a given id.
How to use Spring @PathVariable annotation
http://localhost:9091/student/books/{id}
Let’s see the controller class example using the @PathVariable annotation
@RestController
@RequestMapping("/student")
public class StudentController {
@GetMapping("/{id}")
public Student getStudentUsingPathVariable(@PathVariable String id) {
Student student = new Student();
student.setId(1l);
student.setName("Jon");
student.setRollNumber("0126");
student.setUniversity("RGTU");
if (id != null) {
return student;
}
return null;
}
}
We can test the above API using postman.
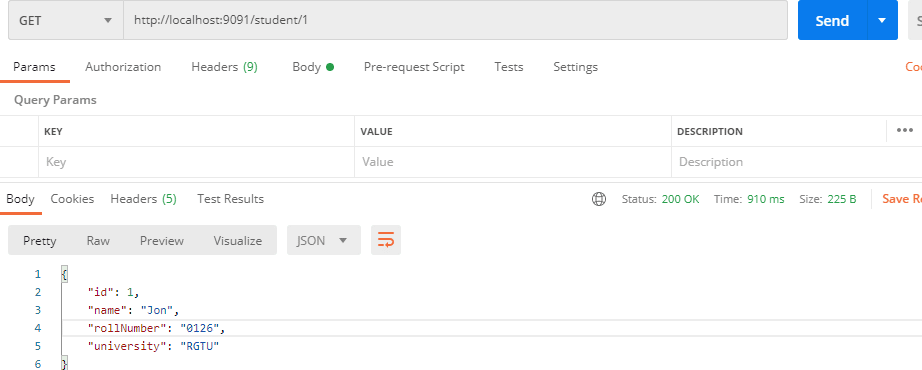
Let’s see how to define the rest endpoint using @RequestParam
annotation.
How to use Spring @RequestParam annotation
While using @RequestParam we send data as a query string.
http://localhost:9091/student?id=1
@RestController
@RequestMapping("/student")
public class StudentController {
@GetMapping
public Student getStudentUsingRequestParam(@RequestParam String id) {
Student student = new Student();
student.setId(1l);
student.setName("Jon");
student.setRollNumber("0126");
student.setUniversity("RGTU");
if (id != null) {
return student;
}
return null;
}
}
we can test the above endpoint using postman.

Basic points about @PathVariable and @RequestParam annotations.
@PathVariable annotation-
- This annotation was introduced in Spring 3.0, available in org.springframework.web.bind.annotation package.
- Optional elements ( name, required, value).
- This annotation used as a method parameter.
- It takes placeholder value from URI.
- The example of rest URI when we use @PathVariable – http://localhost:9093/rest/listofbooks/{bookId}
@RequestaParam annotation-
- This annotation introduced in Spring 2.5, available in org.springframework.web.bind.annotation package.
- Optional elements (name, required, value).
- This annotation used as a method parameter.
- It takes parameter value from URI.
- The example of rest URI when we use @RequestParam –
– http://localhost:9093/rest/listofbooks/{bookId}/book?bookName=book1
@PathVariable annotations Example in Spring Boot.
prerequisites –
- JDK 1.8
- Eclipse
- maven
- postman
Create maven project, Don’t forget to check ‘Create a simple project (skip)’click on next. Fill all details(GroupId – pathvariableexample, ArtifactId – pathvariableexample and name – pathvariableexample) and click on finish. Keep packaging as the jar.
Modify the pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>pathvariableexample</groupId> <artifactId>pathvariableexample</artifactId> <version>0.0.1-SNAPSHOT</version> <name>pathvariableexample</name> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.2.RELEASE</version> </parent> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> </project>
Define classes.
Book.java
package pathvariableexample; public class Book { int bookId; String bookName; String bookPrice; public int getBookId() { return bookId; } public void setBookId(int bookId) { this.bookId = bookId; } public String getBookName() { return bookName; } public void setBookName(String bookName) { this.bookName = bookName; } public String getBookPrice() { return bookPrice; } public void setBookPrice(String bookPrice) { this.bookPrice = bookPrice; } }
BookController.java
package pathvariableexample; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; import java.util.*; @RestController @RequestMapping(value = "/rest") public class BookController { @RequestMapping(value = "/book/{bookId}",method = RequestMethod.GET) public Book getBookById(@PathVariable int bookId) { List<Book> listBook = createBook(); for(Book book : listBook) { if(book.getBookId() == 1) { return book; } } return null; } public List<Book> createBook() { Book book = new Book(); book.setBookId(1); book.setBookName("book1"); book.setBookPrice("100"); Book book1 = new Book(); book1.setBookId(2); book1.setBookName("book2"); book1.setBookPrice("200"); List<Book> bookList = new ArrayList<Book>(); bookList.add(book); bookList.add(book1); return bookList; } }
SpringMain.java
package pathvariableexample; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.ConfigurableApplicationContext; @SpringBootApplication public class SpringMain { public static void main(final String[] args) { final ConfigurableApplicationContext configurableApplicationContext = SpringApplication .run(SpringMain.class, args); } }
If you encounter any port related issue, define application.properties and change the port.
application.properties
server.port = 9093
Run the SpringMain class and deploy the server.
Test the rest URI.

@RequestParam example in Spring Boot.
Let’s modify the controller class.
BookController.java
package pathvariableexample; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController; import java.util.*; @RestController @RequestMapping(value = "/rest") public class BookController { @RequestMapping(value = "/listofbooks/{bookId}/book",method = RequestMethod.GET) public Book getBookById( @PathVariable int bookId, @RequestParam String bookName) { List<Book> listBook = createBook(); for(Book book : listBook) { System.out.println("bookIs is ---"+bookId); System.out.println("bookName is ---"+bookName); if(book.getBookId() == 1 && book.getBookName().equals(bookName)) { return book; } } return null; } public List<Book> createBook() { Book book = new Book(); book.setBookId(1); book.setBookName("book1"); book.setBookPrice("100"); Book book1 = new Book(); book1.setBookId(2); book1.setBookName("book2"); book1.setBookPrice("200"); List<Book> bookList = new ArrayList<Book>(); bookList.add(book); bookList.add(book1); return bookList; } }

That’s all about @PathVariable and @RequestParam annotations in Spring Boot. You may like.
- @RestController and @Controller annotation example in Spring Boot.
- @RequestMapping annotation example In Spring Boot.
- @RequestBody and @ResponseBody annotation example in Spring Boot.
- @RequestHeader annotation example by using Spring Boot.
- @SpringBootApplication annotation example in Spring Boot.
- @Component, @Controller, @Service and @Repository annotations example using Spring Boot.
- @Configuration annotation example using spring boot.
- @ComponentScan example in spring boot.
- @Transactional noRollbackForClassName example using spring boot
- @Transactional rollbackForClassName example using spring boot
- @Transactional readonly true example in spring boot
- @Transactional noRollbackFor example using spring boot
- @Transactional rollbackFor example using spring boot.
- Jboss 7 EPA datasource configuration using oracle and spring boot.
- Deploy Spring Boot application on external Tomcat.
- Deploy multiple war files in JBoss to different port.
- Spring boot datasource configuration using tomcat
- Spring Boot interceptor example.
- Filter example in Spring Boot.
@PathVariable docs.