In this post we will see the difference between get() and load() in Hibernate with example.
First, we will see the difference between the get() and load() in tabular form. Later we will see all points in detail. Also, we will see complete example Hibernate Session get() and load() methods.
Note – Both get() and load() methods are used to retrieve a row from the database for the given identifier, available in the Session interface. Internally both use first-level caches.
Also, The object returned by a get() or load() method is persistent. Check docs here.
Get() | Load() |
As soon we call the get() method, it will hit the database and return the original object. | The load() method returns a proxy object. It will not hit the database until we try to use that object(for example – access some property from the proxy object). |
It returns null if no record is there in a database for the given identifier. | It throws ObjectNotFound exception if no record is there in the database for a given identifier(if we try to access some property from the proxy object then only it will throw an exception). |
Let’s see both points in details.
The get() method returns original object but load() methods retruns proxy object.
The get() method hit the database and returns a real object that contains all field that is initialized with the proper value. In the case of load(), we will have a student object but all fields initialized with null since the load() method returns a proxy object.
We have saved a student object in the database and trying to retrieve that record using the get() and load() method. Let’s see how do student object look like in both cases.
Using Session get() method –

In case of get() method, all fields of student object has been initialized with proper value.
Using Session load() method –

Observe the above screenshot, in case of load() method all field is initialized with the null value. At this point, no SQL query has been fired.
If no record exists in database.
If there is no record for given identifier get() method returns null.
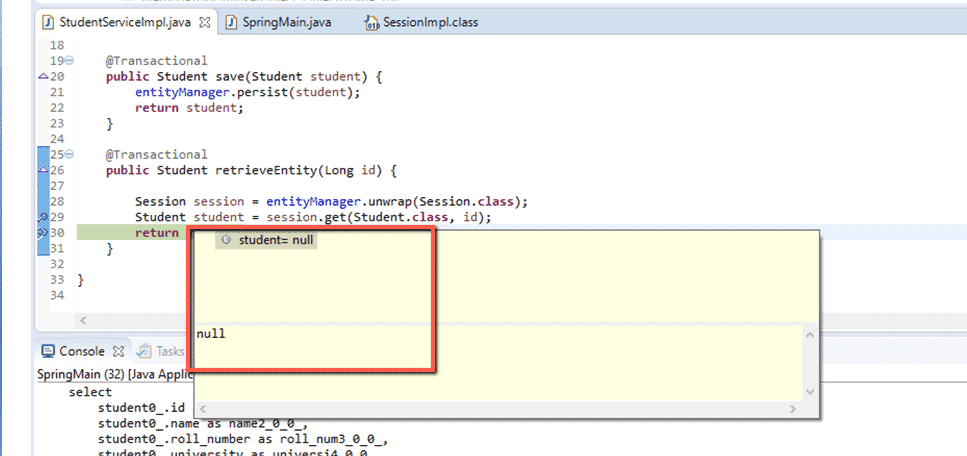
The load() method throws EntityNotFoundException.
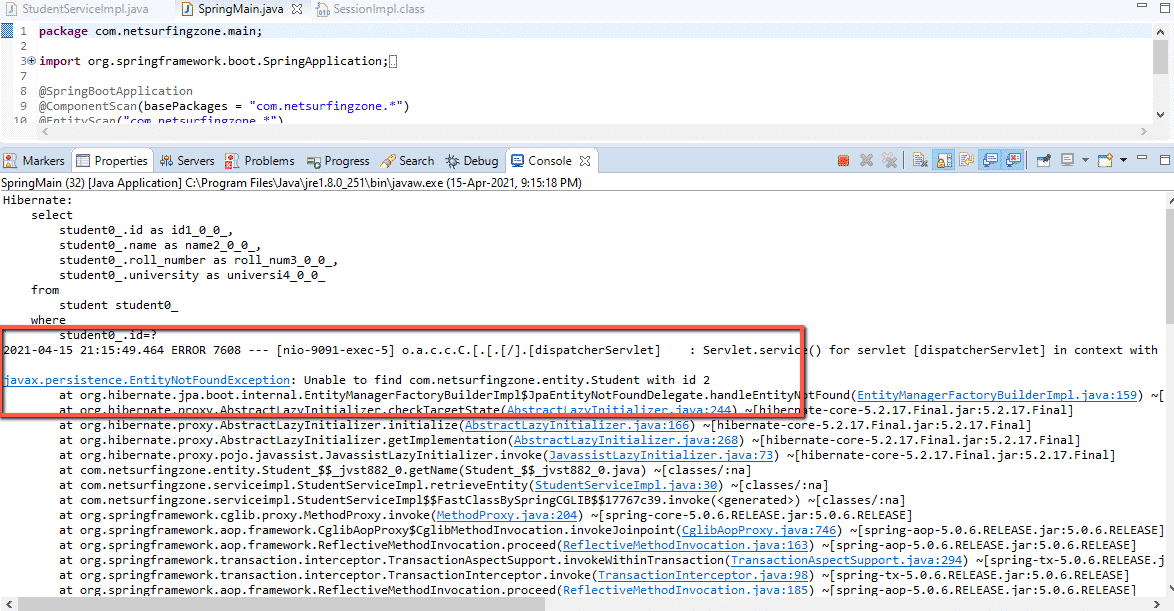
Let’s see an example that demonstrate difference between get() and load() in Hibernate.
create maven project, Don’t forget to check ‘Create a simple project (skip)’ click on next. Fill all details(GroupId – hibernategetvsload, ArtifactId – hibernategetvsload, and name – hibernategetvsload) and click on finish. Keep packaging as the jar.
Add maven dependency.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>hibernategetvsload</groupId>
<artifactId>hibernategetvsload</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>hibernategetvsload</name>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.2.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
</dependencies>
</project>
The directory structure of the application.
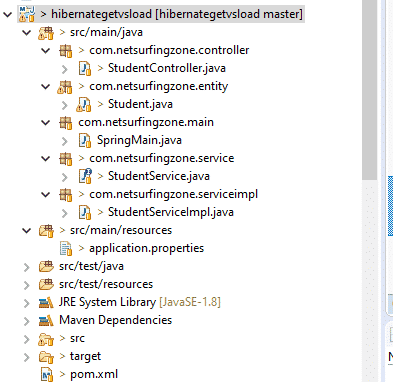
Student.java
package com.javatute.entity;
import java.io.Serializable;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
@Entity
@JsonIgnoreProperties({"hibernateLazyInitializer", "handler"})
public class Student implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "roll_number")
private String rollNumber;
@Column(name = "university")
private String university;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getRollNumber() {
return rollNumber;
}
public void setRollNumber(String rollNumber) {
this.rollNumber = rollNumber;
}
public String getUniversity() {
return university;
}
public void setUniversity(String university) {
this.university = university;
}
}
StudentService.java
package com.javatute.service;
import org.springframework.stereotype.Component;
import com.javatute.entity.Student;
@Component
public interface StudentService {
public Student save(Student student);
public Student retrieveEntity(Long id);
}
StudentServiceImpl.java
package com.javatute.serviceimpl;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.hibernate.Session;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import com.javatute.entity.Student;
import com.javatute.service.StudentService;
@Service
public class StudentServiceImpl implements StudentService {
@PersistenceContext
private EntityManager entityManager;
@Transactional
public Student save(Student student) {
entityManager.persist(student);
return student;
}
@Transactional
public Student retrieveEntity(Long id) {
Session session = entityManager.unwrap(Session.class);
Student student = session.get(Student.class, id);
//Student student = session.load(Student.class, id);
System.out.println("sql query will not print immediatly in case of load() method ");
return student;
}
}
StudentController.java
package com.javatute.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.javatute.entity.Student;
import com.javatute.service.StudentService;
@RestController
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@PostMapping("/create")
public Student createStudent(@RequestBody Student student) {
Student createResponse = studentService.save(student);
return createResponse;
}
@GetMapping("/retrieve/{id}")
public Student getStudent(@PathVariable Long id) {
Student response = studentService.retrieveEntity(id);
return response;
}
}
SpringMain.java
package com.javatute.main;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.domain.EntityScan;
import org.springframework.context.annotation.ComponentScan;
@SpringBootApplication
@ComponentScan(basePackages = "com.javatute.*")
@EntityScan("com.javatute.*")
public class SpringMain {
public static void main(String[] args) {
SpringApplication.run(SpringMain.class, args);
}
}
application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/springbootcrudexample
spring.datasource.username=root
spring.datasource.password=root
spring.jpa.hibernate.ddl-auto=create
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.format_sql=true
server.port = 9091
#logging.level.org.hibernate.type.descriptor.sql=trace
See more details about Session and SessionFactory here.
That’s all about difference between get() and load() in Hibernate.
See Hibernate Session get() and load() docs here.
Download example from github.
You may like other hibernate tutorial.
- @Version Annotation Example In Hibernate.
- Session and Session factory in hibernate.
- Hibernate Validator Constraints Example Using Spring Boot.
- @Temporal Annotation Example In Hibernate/Jpa Using Spring Boot.
- Hibernate Table Per Concrete Class Spring Boot.
- Hibernate Table Per Subclass Inheritance Spring Boot.
- Hibernate Single Table Inheritance using Spring Boot.
- One To One Mapping Annotation Example in Hibernate/JPA using Spring Boot and Oracle.
- One To One Bidirectional Mapping Example In Hibernate/JPA Using Spring Boot and Oracle.
- One To Many Mapping Annotation Example In Hibernate/JPA Using Spring Boot And Oracle.
- Many To One Unidirectional Mapping In Hibernate/JPA Annotation Example Using Spring Boot and Oracle.
- One To Many Bidirectional Mapping In Hibernate/JPA Annotation Example Using Spring Boot and Oracle.
- Many To Many Mapping Annotation Example In Hibernate/JPA Using Spring Boot.