Understanding the difference between collection, Collections, and Collection in Java.
A collection is an object that groups one or more than one element into a single unit. Collection framework provides interfaces and classes to store, retrieve and manipulate the data.
A collection framework contains interfaces, implementations, and algorithms.
interfaces -Interfaces allow collections to be manipulated independently of the details of their representation
implementations- These are reusable data structures.
algorithms – These are the methods that perform a different operation such as searching and sorting, on objects that implement collection interfaces.
Algorithms are reusable functionality.
Use of collection:-
- We can add objects to the collection.
- We can remove objects from the collection.
- We can find out an object or group of objects from the collection.
- We can iterate through the collection.
[stextbox id=’info’]Don’t get confuse :-
Don’t get confused about collection, Collection, Collections. Let’s see the difference between them.
collection:-it represents any of data structure in which objects are stored and iterated over.
Collection:-This is an interface available in java.util package.
Collections:- This is a class available in java.util package. It has a static utility method for use with collections.[/stextbox]
Collection hierarchy in Java.
Here we will see the collection hierarchy in java, what are the important classes and interfaces available in collection framework.
collection hierarchy in java –
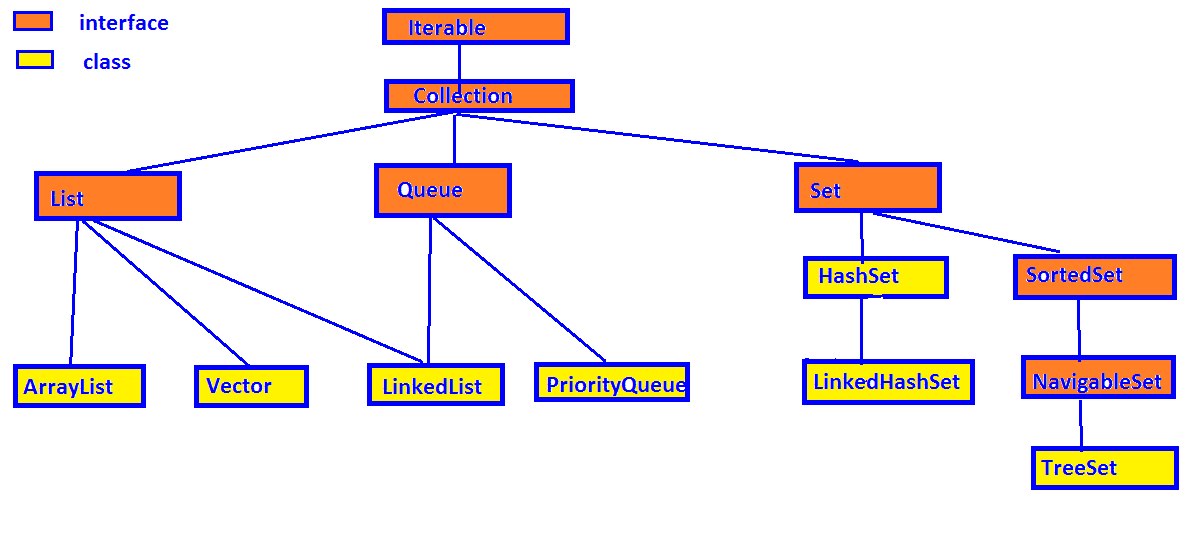
Since Map is not part of Collection interface we will see it separately.
Sometimes you might encounter this question in the interview, is Map part of the collection hierarchy? The answer is no. Map doesn’t extend Collection interface like List and Set. Map is an interface which available in util package, which has its separate hierarchy.

Don’t forget Collections class –
Collections is a class available in util package, which has static util methods.

Collections class in Java with example
Collections is a class, available in util package which extends Object class whereas Collection is an interface. Collections class contains static util methods, which we can directly use in our application. Let’s see a couple of methods which we use frequently.
Collections.sort() –
Suppose we have a String type list, having a couple of elements. We want to sort it using sort() method.
import java.util.ArrayList; import java.util.Collections; import java.util.List; public class CollectionsExample { public static void main(String[] args) { // creating a list object List<String> listOfstring = new ArrayList<>(); // adding some element in list // for beginners it is important to understand we have created a list // listOfstring, but as of now it empty listOfstring.add("ram"); listOfstring.add("mohan"); listOfstring.add("sohan"); listOfstring.add("aman"); listOfstring.add("deep"); System.out.println("before Collection.sort() ---- " + listOfstring); // Since sort() is static method we can call with class name directly Collections.sort(listOfstring); System.out.println("after Collection.sort() ---- " + listOfstring); } }
Output of the above program is –
before Collection.sort() —- [ram, mohan, sohan, aman, deep]
after Collection.sort() —- [aman, deep, mohan, ram, sohan]
sort() method has two overloaded version. First one takes a single parameter – sort(List<T> list), another one takes two parameters something like – sort(List<T> list, Comparator<? super T> c). This overloaded version used for sorting purpose, when we sort the list object using a comparator. For more details please visit this post.
Collections.swap() method in Java.
swap(List<?> list, int i, int j)
This method is used to swaps the elements at the specified positions in the specified list.
Please visit java doc for more collections class methods.
Difference between Arrays and Collections Framework in Java. Benefits of collections over arrays in java.
In this section we will see the difference between Arrays and Collections Framework in Java with example.
Sr. No. | Arrays | Collection Framework |
---|---|---|
1. | Arrays are fixed in size. | Collections are growable in nature . |
3. | Since arrays are static in nature, for memory perspective arrays are not recommended to use. | Collections classes are dynamic in nature , for memory perspective collections are recommended to use. |
4. | Arrays are better than collections in performance. | Collections are not poor in performance than Arrays. |
5. | Arrays does't have predefined class which can remove duplicacy or can sort the elements. | Collections have predefined classes which can be use for different purpose. |
6. | Array can contains both primitives and object type. | Collections can hold only objects but not primitive. |
7. | Arrays doesn't have any API like Map. | We have Map in collections(Although Map is not part of Collection interface). |
8. | If we know the size of elements then it is good to go for arrays. | if we are not sure about size we will go for Collections. |
Let’s see all points in details.
Arrays are static in nature whereas Collections are dynamic in nature.
Arrays are static in nature, once we define the size of arrays we can’t change it.
public class ArraysExample { public static void main(String[] args) { int[] intArray = new int[2]; intArray[0] = 10; intArray[1] = 11; for (int a : intArray) { System.out.println(a); } } }
Output is –
10
11
In the above program, we can only insert two elements as we have declared the size of intArray is two. So what will happen, if we try to insert the third element in array intArray, there is no compilation error but at runtime, it will throw java.lang.ArrayIndexOutOfBoundsException.
public class ArraysExample { public static void main(String[] args) { int[] intArray = new int[2]; intArray[0] = 10; intArray[1] = 11; intArray[2] = 11; for (int a : intArray) { System.out.println(a); } } }
Above program will throw an exception at runtime.
Exception in thread “main” java.lang.ArrayIndexOutOfBoundsException: 2
at ArraysExample.main(ArraysExample.java:7)
Memory in case of Arrays and Collections-
Collections have classes which are dynamic in nature. For example, in ArrayList, we can insert any number of elements.
import java.util.ArrayList; import java.util.List; public class ArraListExample { public static void main(String[] args) { List<String> listObject = new ArrayList<>(); listObject.add("ram"); listObject.add("mohan"); listObject.add("shyam"); listObject.add("mohan"); listObject.add("ram"); // you can add some more element listObject.add("ram"); listObject.add("ram"); System.out.println(listObject); } }
Output is – [ram, mohan, shyam, mohan, ram, ram, ram]
Let’s see why arrays are not recommended to use for memory perspective.
Suppose we are declaring an array with size 100, but later we have only 4 elements. In this case JVM will allocate memory for 100 elements, the memory for the rest of the element will be waste only. In below program, we will have 10,11,11,11 in output but also it will print 0, 96 times(since default value of int is zero)
public class ArraysExample { public static void main(String[] args) { int[] intArray = new int[100]; intArray[0] = 10; intArray[1] = 11; intArray[2] = 11; intArray[3] = 11; for (int a : intArray) { System.out.println(a); } } }
Output is –
10
11
11
11
0
0
0
.
.
.
0
In the case of collection classes, there is not this kind of issue.
Performance in case of Arrays and Collections –
Arrays are better in performance than Collections. For example, in the case of ArrayList, we have add() method where we can add n number of elements but internally add() method used nothing but arrays. If you look into add() method we have something like
elementData = Arrays.copyOf(elementData, newCapacity);
Here we can see we are using copyOf() method which will copy the old data to a new data. What if we have a large number of elements in our list, yes it’s will affect performance. But still, ArrayList is very popular for real-time programming Please visit this post for how to define custom ArrayList.
Predefined API in case of Arrays and Collections –
Arrays have less predefined API, whereas Collections have a lot of classes and interfaces. we have ArrayList, Vector, LinkedList, HashSet, TreeSet and many more. This is the one of core benefit of Collections over arrays. These classes have been designed in such a way that we can directly use for the different purpose, no need to define custom logic. For example, if we don’t want to allow duplicate elements in our Collections we can go for Set.
Arrays can have both primitives or Object but Collections can have only objects –
We can define arrays type of primitives as well as Object, but Collections can have the only Object type. Let’s see the example –
public class ArraysExample { public static void main(String[] args) { // declaring int array as primitives int[] intArrayAsPrimt = new int[2]; intArrayAsPrimt[0] = 10; intArrayAsPrimt[1] = 11; // declaring int array as Objects Integer[] intArrayAsObj = new Integer[2]; intArrayAsObj[0] = 20; intArrayAsObj[1] = 31; for (int a : intArrayAsPrimt) { System.out.println("declaring int array as primitives " + a); } for (int a : intArrayAsObj) { System.out.println("declaring int array as Objects " + a); } } }
Output is –
declaring int array as primitives 10
declaring int array as primitives 11
declaring int array as Objects 20
declaring int array as Objects 31
In case of collections, we have always Objects –
import java.util.ArrayList; import java.util.List; public class CollectionsExample { public static void main(String[] args) { // creating a list object of String type List<Integer> listOfNumb = new ArrayList<>(); listOfNumb.add(10); listOfNumb.add(20); listOfNumb.add(30); listOfNumb.add(40); System.out.println(listOfNumb); //we can't do something like below //List<int> listOfNumb = new ArrayList<>(); } }
Other Collection examples in Java.
- ArrayList in Java.
- Vector in Java.
- LinkedList in Java.
- HashSet in Java.
- LinkedHashSet in Java.
- TreeSet in Java.
- HashMap in java.
- Hashtable in java.
- LinkedHashMap in Java.
- TreeMap in java.
- How to avoid duplicate elements in ArrayList.
- Difference between ArrayList and LinkedList in java.
- Difference between List and Set in java.
- Difference between HashSet and TreeSet in java.
- Difference between HashSet and HashMap in java.
- Difference between Enumeration and Iteration in java.
- Difference between Iterator and ListIterator.
- Difference between Comparable and Comparator in java.
- Difference between HashMap and Hashtable in java.
- Comparable in java? Program to sort on basis of id using Comparable.
- Comparator in java. Program to sort using Comparator.
- Create custom HashSet in java.
- How to create a custom linked list in java
- How to allow duplicate elements in Set
- How to define custom ArrayList in java
- How to create a custom linked list in java
- How to create custom HashSet in Java.
- How HashSet works internally in java
- How get method of ArrayList work internally in java.
- Fail fast and fail safe example in java.
That’s all about Collection in Java with Example.